Introduction:
With so much information, entertainment, and educational material online, watching videos is a great way to learn more about specific topics of interest. However, it can be hard to watch these videos if you happen to have a slow or intermittent internet connection, as it can cause videos to buffer or lag.

That’s why in this article we are going to be using Python to create a YouTube video downloader that can be used to download our favourite YouTube videos directly to your computer. This will allow you to watch them directly from your device, making them accessible at all times. Whether you are new to Python or have some prior experience, this project is a great way to learn more about making functional programs and helps you apply your knowledge in a practical and fun way, and is a great beginner Python project for kids.
Who is this Project For?
This is an intermediate-level project for those who are new to Python. At this point, you should already know the basics of creating functions, installing packages, and creating simple GUIs (graphical user interfaces) with Tkinter. These are the concepts that you will use and build upon as you code this project.
What Will We Learn?
This video downloader project focuses on using the Tkinter library to create the program’s GUI, as well as the Pytube library to download videos directly from YouTube. Both of these libraries are very versatile and can be used for a wide variety of projects, making this project a great way to learn new skills that can be used later on for even more projects.
Features to Consider:
- The user should be able to insert any YouTube video link to download.
- The video should be downloaded and saved to the computer.
- The GUI will display a message once the download is complete to prevent users from unintentionally downloading the same file many times.
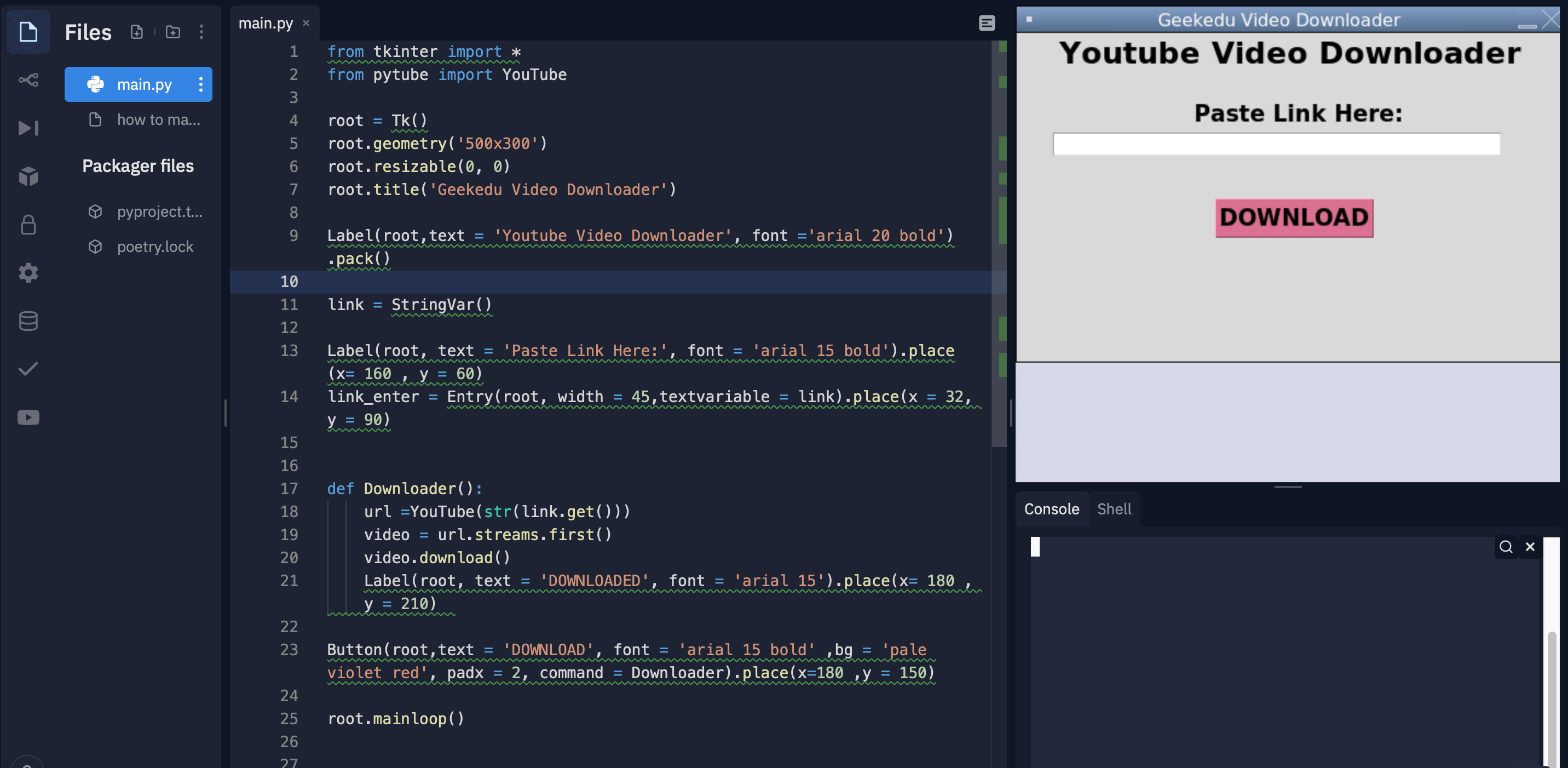
Main Steps:
This project can be broken down into 4 main steps:
- Import and setup modules
- Create the main Tkinter GUI
- Define the Downloader function
- Create the GUI’s ‘Download’ button
Step 1: Import the Modules:
The first thing we’ll need to do to begin the project is import all of the libraries and modules that we’ll be using. Because the Tkinter and Pytube libraries are non-native, meaning that they must be installed and imported to the computer before being used. The first step we will need to do is install these libraries on the device. To do this, you can open the command line on your computer and type the command:
Now that the libraries have been set up, it’s time to import them into the program. This will let the program know that we will be using these libraries. The code for this step will look like this:
Step 2: Create the main Tkinter GUI
Now that all of the modules have been imported, it’s time to create the program’s graphical user interface. This will involve setting up the canvas and its dimensions, titling the window, and adding a text input box. To begin, we’ll initialize Tkinter and create the window. Our window will be 500x300 pixels so we will set these as the dimensions. After this is done, the code will be:
Now we’ll need to set up the window so that it cannot be resized. This will prevent the elements such as titles, buttons, and text inputs from being shifted around and resized. We will also title the window so that users know exactly what it’s called. In this case, we’re titling the window “Geekedu Video Downloader”. Once this step is complete, the code should look like this:
Next, it’s time to create labels for the GUI, as well as create the text input box. We’ll start by creating a label to let users know what the program does. To do this, we will create a label that says “YouTube Video Downloader” and place it at the top center of the window. Underneath, we will also create another label that says “Paste Link Here:” to let users know where to insert the video link. The code for this step of the project will be:
Hint: There is a set format that is used for creating labels with the Tkinter GUI. The format is as follows: Label(root, text = 'Add Label Text Here', font = 'font font size style').place(x= X-coordinate , y = Y-coordinate). Note that any section in bold will be replaced with the desired text, format, or coordinates for the label.
Now that the labels for the GUI are complete, it’s time to add the text input box. To start, we’ll create a variable called “link”, and set it to a blank string variable.
Hint: This will let the program know that the text entered into the text box will be a string, and the computer can take this information and store it as a variable.
Next, we’ll create a text box where users can paste their video’s link. This element will follow a very similar format to the labels we created earlier, and in the end, the code will look like this:
Once this step is complete, the main GUI is finished and it’s time to move on to code the main download function of the program.
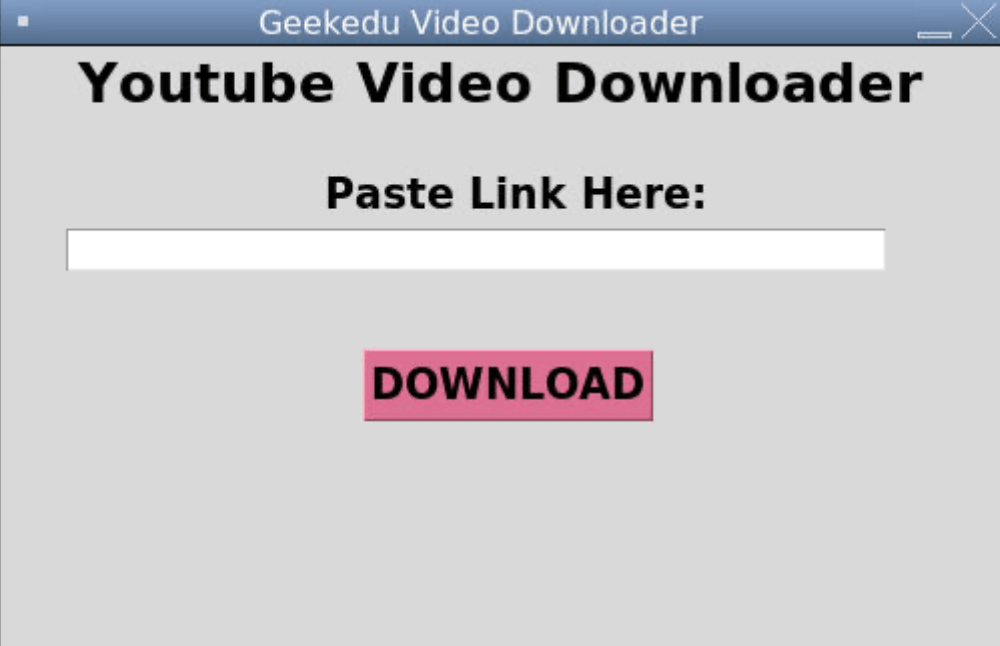
Step 3: Define the Download Function
Now we’ll move on to create the download function. This function will take the link that users input in the text box, download the video, and display the message to let users know that the download was successful. The first section of code for this step of the program will look like this:
As you can see in the code, we’ve started by defining the initial Downloader function. Then, we used two more lines of code to get the user’s input, and essentially let the program know that the link is the video that should be downloaded. Finally, we added a line of code to download the video to your device, which will allow you to play the video even without an internet connection.
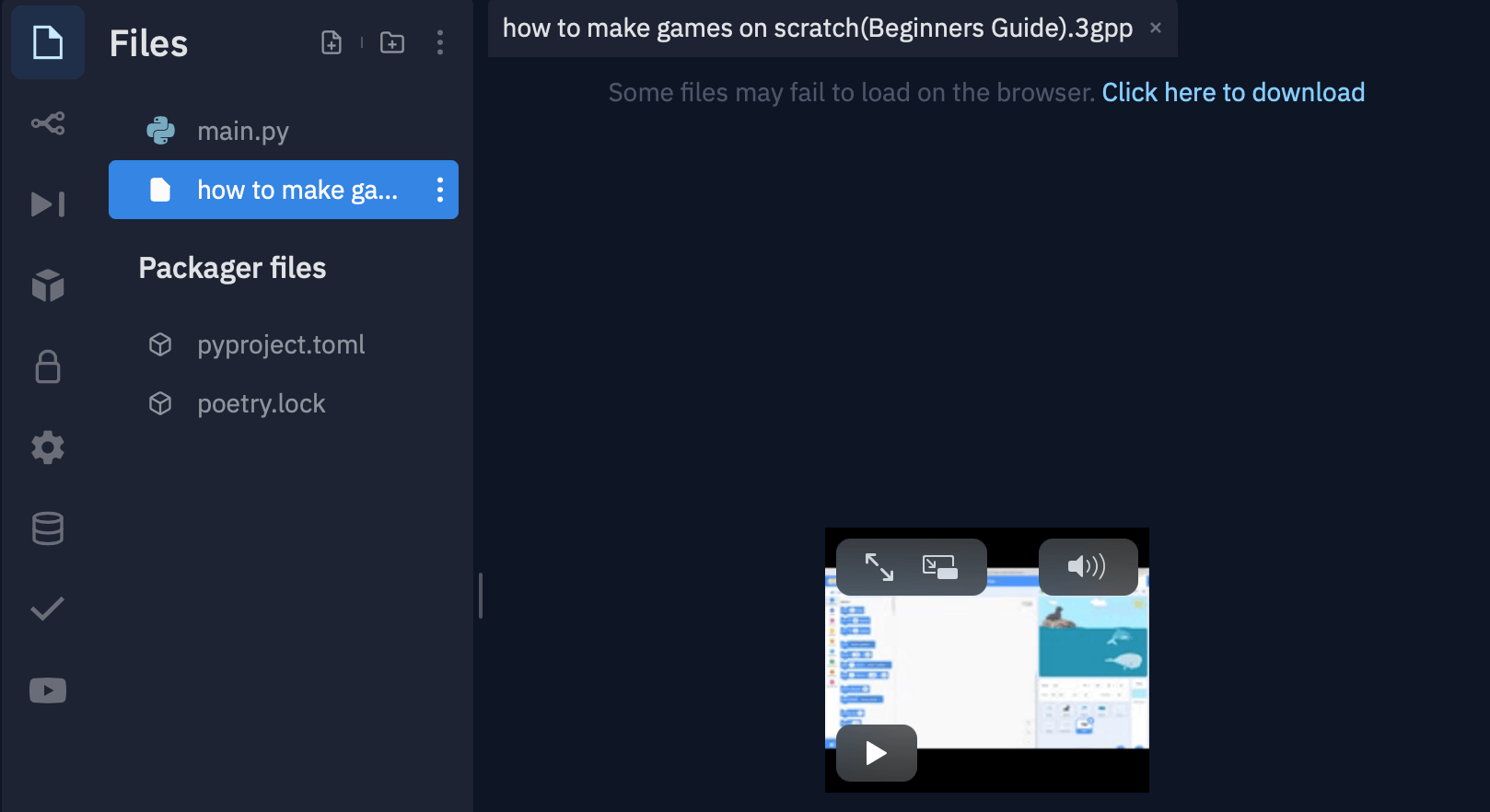
Finally, we need to add the downloaded message. To do this, we will add a label that displays a “Downloaded” message. The code for this step will be:
Step 4: Create the GUI’s ‘Download’ Button
Finally, we just need to add the ‘Download’ button to the GUI, as well as a line of code to run the whole project. We will use the same basic formatting as was previously used for the labels and input box to create a button. Finally, we will use the root.mainloop() command to set up the program and allow it to be run. This command will essentially run the pain Python file and loop it forever, which means the program will always run. Once this final step is complete the code will look like this:

Hint: Note that in the line of code where we set up the Download button, the format is slightly different as we added colour as well as padding and a command. Here are the main elements of this formatting:
- bg: This section of the formatting sets the button’s background colour- the colour that it will be filled with.
- padx: This section of the formatting determines the padding in the X-direction. This means that there will be a space along the x-axis that separates the button from other elements. This spacing is determined by the value of the padding.
- command: this section of the formatting defines what function should be run when the button is pressed. In this case, the command is “Downloader”, meaning that the downloader function will be run whenever the button is pressed.
Project Complete!
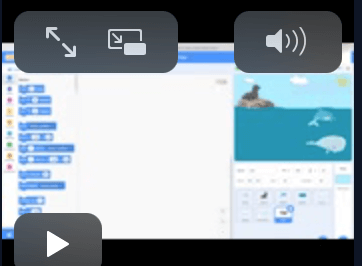
And now the project is complete! At this point, you will be able to use the YouTube video downloader to download videos so you can watch them offline. Hopefully, you’ve enjoyed this beginner Python tutorial for kids, and if you’re stuck or have any issues with your code, try reviewing it again either in your text editor or by looking at the code mentioned above as a reference.