Introduction :
Since the start of the Covid-19 pandemic that has kept many of us at home, it has been statistically proven that the average human being at home, ate more food than they would normally have. For some of us, it could be very clear that we added on a few more pounds. To others, it may not. Regardless of the clarity or idea you might have about your weight change during the pandemic, we have a special formula/tool that can be used to determine our body’s physical health status. It is the Body Mass Index formula. Invented by Adolphe Quetelet in the 19th century, the “BMI” formula uses the height (in meters) and the weight(in kg) of a person and applies a formula. The formula is : weight in kg/height in meters squared. This will return a number which can then be compared and analyzed through a chart like this one :

Or through a more, simpler chart like this one :
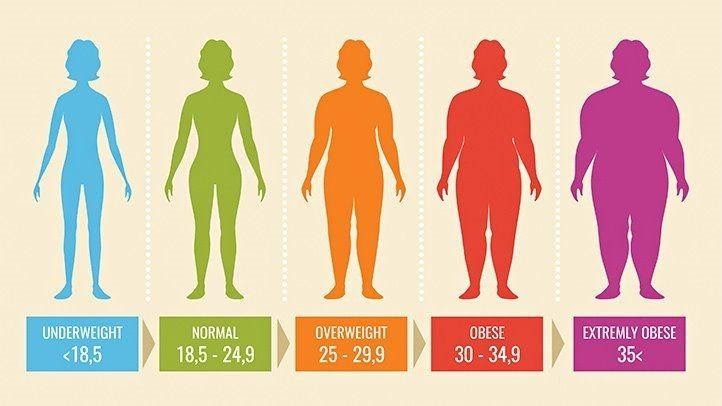
We will use this kind of chart to form our logic which determines the user’s physical wellness and status.
Who Is This Project For ? :
This project is best for anyone who is a beginner or intermediate Pythonista. Many of the concepts featured in this project are concepts you mostly have already come across. If not,
you at least must have worked with beginner concepts such as functions and scoping with variables.
What Will We Learn? :
We will be incorporating many familiar concepts for beginners such as Operators, Data Types, Control Flow statements, and Boolean Operators. We’ll also cover some intermediate concepts in Python such as Object Oriented Programming and Error Handling that we will use with the Tkinter GUI library. If you don’t know any of the intermediate concepts mentioned above, you’ll surely pick them up along the way or at least will get the opportunity to be introduced to them. This also applies to the Tkinter GUI library. If this is your first time working with this library, we suggest you maybe check out Python Project for kids: Python Password
Generator to get a more beginner-friendly introduction to the library. If not, then you’re in the right place! Let’s begin!
Features To Consider :
Here are some of the steps we want to consider and plan, before we build our application:
- The GUI should be user-friendly and easy to use.
- For any reason, if we do not receive any valid input, we should be prepared and not let the program crash.
- We want to give a helpful answer that is useful and makes sense to the user.
- The program should be able to flawlessly apply the BMI formula to the values that were given into the application.
- We want to be able to check the BMI and compare it through the BMI chart to return a reliable BMI physical status.
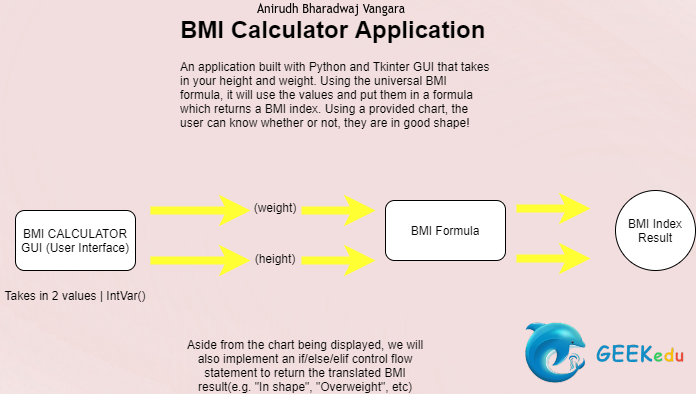
(We will use StringVar() instead of IntVar() to better handle decimals!)

Main Steps:
Step 1 : Initial Setup
Again, we’ll be using Tkinter GUI Library for this project. If you haven't downloaded it already, please do so. If you’re using a fully-fledged Python IDE like PyCharm, then you can skip the downloading process because it’s already downloaded and built-in. Now we want to import it into our project. To do so, type the following :
Now that we’ve officially imported it into our Python project, we want to start to use it right? Let’s start by declaring and creating our project window. We do this by creating a root. Some like to call it a window. Both work but, we will use root as the variable name instead.
We do not want to allow the application window to be resized. We could if we wanted to by setting both values to True, True but it will hurt the originality of the GUI and application.
Step 2 : Defining The Variables
Great! We’ve created the window, we want to go ahead and define some variables for our project. These will be global variables so that we can use them anywhere in our project. The first 2 variables we will create are for storing the input that the user gives; the height and the weight. The next 2 variables are for later on in the project. The bmi_value variable is for the floating number we get once we’ve applied the formula. The bmi_status is the variable that will store the physical status that is returned after the BMI value is filtered through the control flow logic we will implement later on.
Step 3: The BMI Function Logic
Here comes the challenging part of our application; the BMI function and its logic. Below are the steps we need to cover to make this section:
- We need to first understand the formula
- We need to then accept the arguments and apply the formula to them
- We need to then return a number which should be the BMI
- Using the returned BMI, we want to send it through a series of if/else/elif statements that will determine and return a physical wellness status of the user based on their BMI and the BMI chart guidelines.
So we will declare the function and have *args as the parameter. *args will allow any arbitrary number of arguments into our function. This is a good idea if you aren’t 100% sure how many arguments will be passed into the function.
Now, remember when we declared the variables as StringVar()? That would mean, the value passed in would be a string. Once it is passed in, we will convert the string value into a float. But what if a series of letters were passed in, through the input field instead of numbers?
Words cannot be converted into integers! So if this was the case, we would get a ValueError from Python, and our program would crash! So we have to be smart and be prepared for this error. To handle it, we will use Try and Except. So, if the function will try to attempt the logic and program we have coded. If it does not work, it will except it and not allow the program to crash.
Alright! We’ve set up an error handling program so we can now focus on the logic part of the program. The first thing we want to do is retrieve the values from the global variables we declared earlier that currently are storing variable values of the height and weight of a user. Because they are in string format, we cannot directly work with them. Instead, we can convert them into a float and store them in local variables; height and weight. Now that they are floats, we can apply arithmetic operators to them. In other words, we can apply the BMI formula to the values.
Once we’ve stored the result in the local bmi variable, let’s store it in the empty global variable we created earlier, bmi_value.
TIP : To make sure the BMI doesn’t have over 2 decimal places, use f"Your BMI is {bmi:.2f}". This kind of string formatting will limit the number of decimal places that can be displayed.
Here comes the second part of the function- determining the physical wellness status of the user. We can do this with this chart :
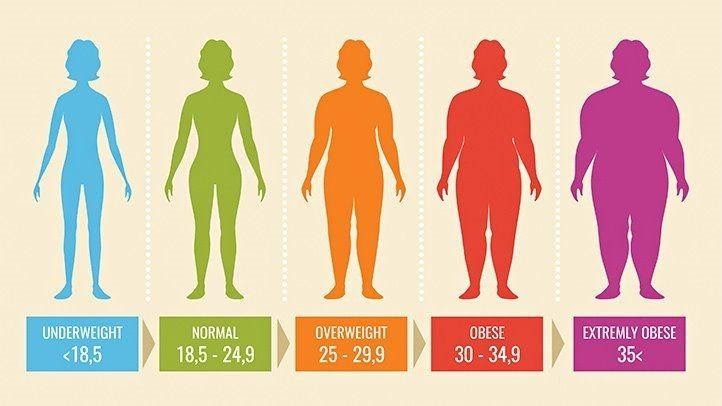
We can fulfill this requirement with the help of control flow statements and if/else/elif conditions.
(This goes inside the function)
Now we want to set the value of the local bmi_state variable to the empty global variable, bmi_status. We can do this with :
Great! We’ve officially completed our BMI Function and it’s logic. This is how the function should look like :
Step 4 : Creating The Tkinter GUI
We’ve completed working with the backend of the application. Now we must create the frontend and a UI for the user to input information for our application to work. Below is the code needed for the frontend. If you’ve used Tkinter before, many of the syntax and code used below may be familiar. If not, don’t worry! The comments in the code will help guide you and understand what the code does :
This is a preview of how it should look like, after calculating the BMI :
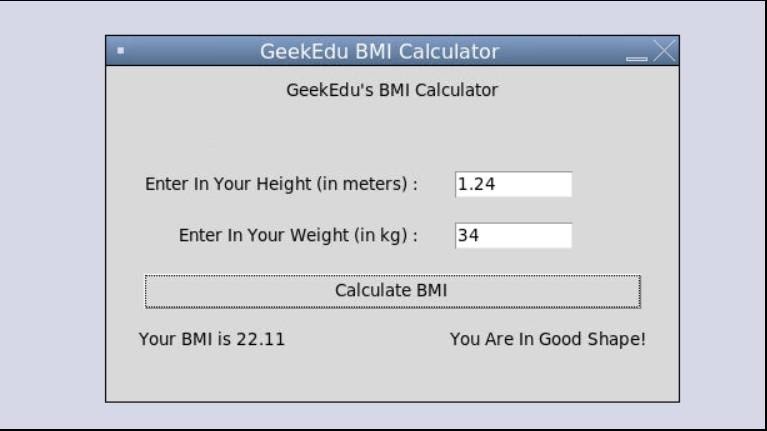
Step 5 : Displaying The BMI!
To display the BMI, we will use 2 labels and have their textvariable attribute, set to the variables we have stored our user’s BMI information in.
Here’s an example of how a sample result should be displayed :
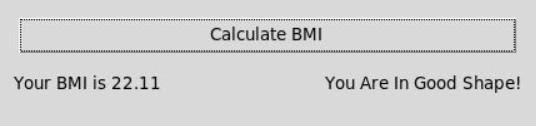
BONUS STEP : MORE INTERACTIVITY!
What if we could make our application more interactive for the user!? We could do this with key bindings?! We actually can, thanks to Tkinter’s bind property. So when a user inputs the height first, and then the weight, they can simply hit the Enter key and this will run the application!
HINT : The "<KP_Enter>" represents the Enter key on the traditional keyboard’s numpad. Below is a better reference with a layout of a computer keyboard :
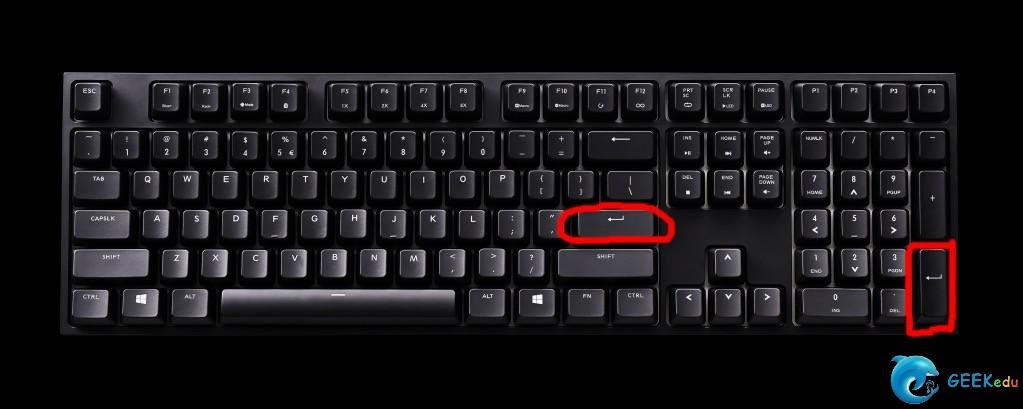