Introduction:
In this article, we are going to be using Python to create a simple Hangman game. This program will ask users to guess a word, and if they get more than 5 answers wrong, they lose..
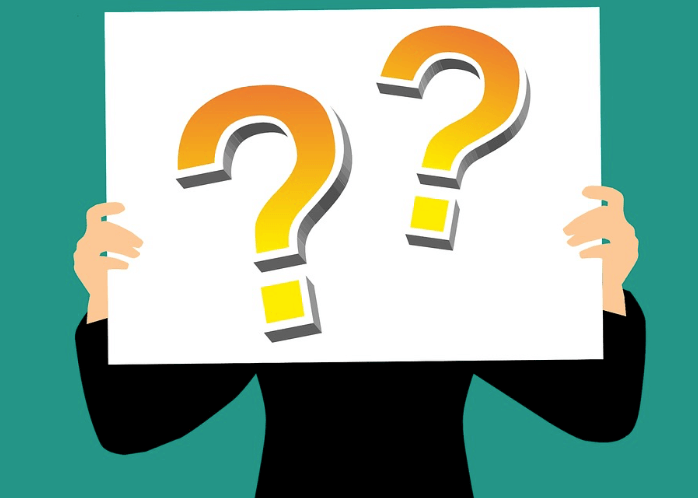
This project is a great way to learn more about making functional programs by creating a functional and versatile program. It’s a great way to build both your creative thinking and problem-solving skills while making a fun and entertaining game as well!
Who is this Project For?
This is an intermediate-level project for those who are new to Python, or those who want a fun way to apply their coding skills. Before starting this project, you should have some experience with creating and using functions, loops, and conditional statements such as if-statements. These concepts are widely used in any computer program, and your knowledge of these concepts will be developed as you work through this project.
What Will We Learn?
This program focuses on creating a functional Hangman game that users can play on their own or with friends. This teaches concepts such as if-statements, functions, and using operators as well as other essential programming concepts.
Features to Consider:
- The user will be given a word to guess
- They are allowed to guess letters, if they get the letter correct it is revealed
- If the player guesses once, their player is drawn on the screen
- If the player gets 5 incorrect guesses, the player has lost and the game is over.
Pseudo Code:
The pseudocode for this project is included below:

Main Steps:
This project can be broken down into 3 main steps:
- Create the main function
- Check user input
- Complete the hangman function
Step 1: Create the Main Function
The first thing we’ll need to do when creating this program is to import the time library. This will allow the program to manage any delay the program may have. We will also import the random module, which will be used to randomly select a word for the game.
Then, we’ll need to create our main function, which will involve creating variables and setting up a list of possible words for the player to guess..

Step 2: Check for User Input
Next, we need to create the Hangman function to ask for user input. We will begin this function by referencing many of the global variables that we created in the main function, and then we will ask the user for their input. This code will be done like so:
Now we need to take this input and check if it is valid. We will do this by first checking whether it is a single letter, and then checking whether it is in the word that the player has to guess. This is the rest of the code for this step:
As you can see, we now have created some code that will control whether or not the input is valid, and if it is, this code will reveal a letter.

Step 3: Complete the Hangman Function
Finally, we need to print the hangman illustrations that will be shown if the user guesses a wrong letter. While this section of code is quite long, it is repetitive, so once you complete one section, the rest will be easy to quickly add in. This is the final section of code for this project, and it is as follows:
You will notice that at the bottom of this code we also included a message for when the player loses the game, as well as an if-statement to congratulate them if they guess the word correctly. We also called the main and hangman functions at the end of the code in order to run the game.
Project Complete!
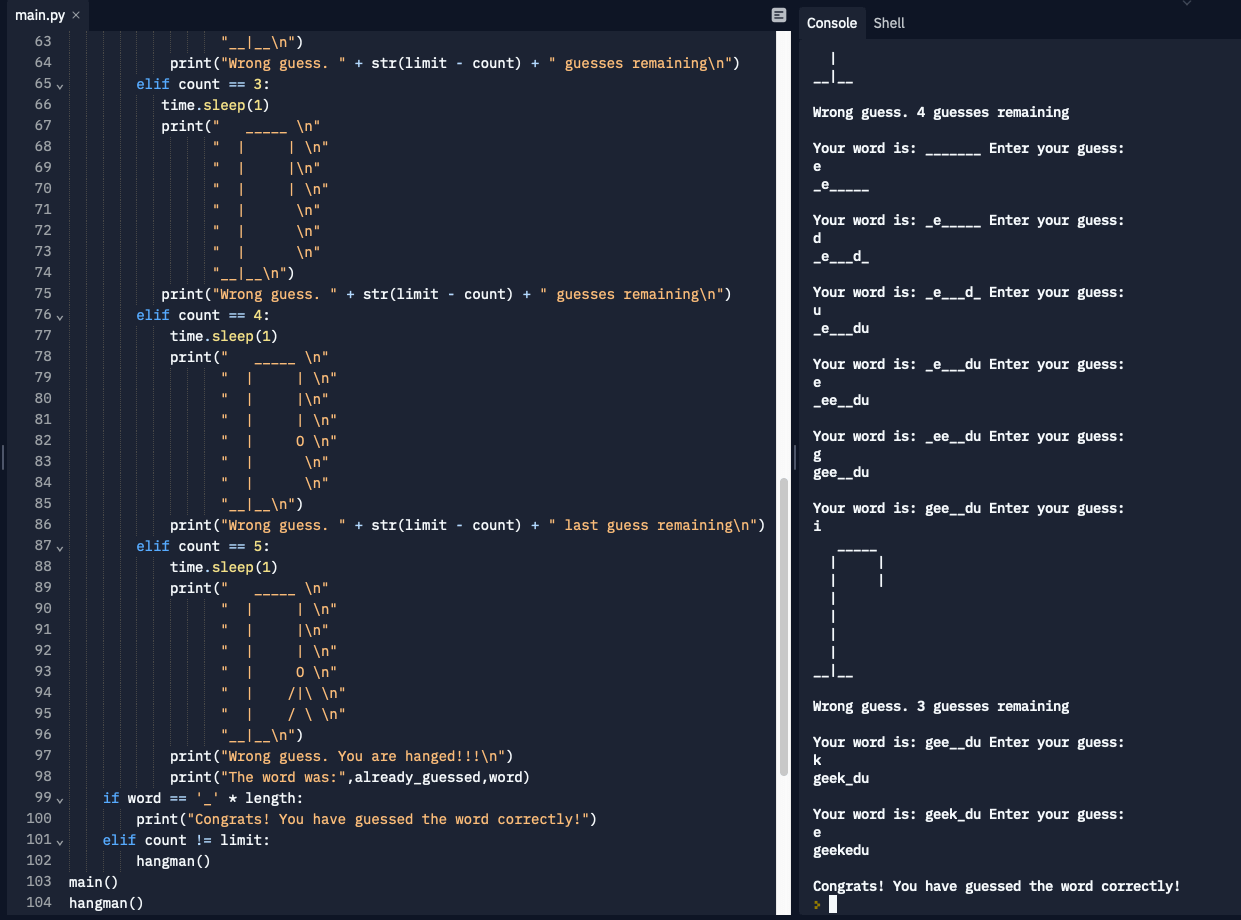
Now the project is complete! We hope that you’ve enjoyed creating this fun and simple hangman game. Feel free to test your code out and check the code mentioned above as a reference if you have any errors.