Introduction:
In this article, we are going to be using some simple Python code to create a simple Snake game. Snake is quite possibly one of the most well-known computer games of all time. It is a simple game that involves maneuvering a “snake” around a screen to pick up “food”. The more the snake gathers, the longer it gets, and the game’s difficulty increases. The game is deceptively simple and is lots of fun to play, and its’ simple code makes it the perfect project for kids or those who are new to Python to create. Regardless of your proficiency with Python, this project is a great way to learn more about making functional programs by creating a fun and simple game to play with friends.

Who is this Project For?
This is a beginner-level project for those who are new to Python. Before starting this project, you should already have experience with functions, if-statements, and the Python Turtle library, as these are the concepts that you will use and build upon as you code this project.
What Will We Learn?
This program focuses on creating simple graphics using Turtle, and creating functions that can be used to control the snake as well as other elements of gameplay. If-statements handle a variety of possible scenarios, and make the game run smoothly. These concepts are essential to know, and will surely be used in just about any Python project.
Features to Consider:
- The snake will be displayed in the main game screen
- Food will appear at a random location within the window
- The snake must touch the food to eat it, and upon doing so, it will get longer
- Upon eating the food, a message will be printed displaying the user’s score, and the food will move to a newly randomized location
- If the snake collides with wither the wall or itself, the game ends
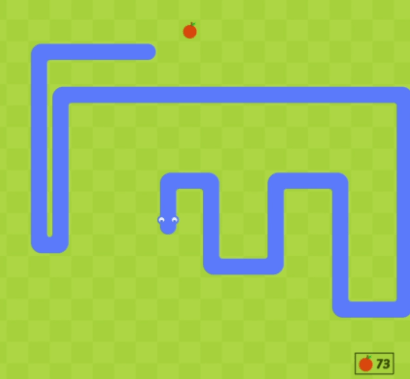
Pseudo Code:
Below is the pseudocode for the snake program:
Main Steps:
This project can be broken down into 4 main steps:
- Import libraries and set up variables
- Create the change and inside functions
- Create the move function
- Finish program setup
Step 1: Import Libraries and Set Up Variables
The first thing we’ll need to do when creating this game is import all of the required libraries. For this program, we will be using the Turtle library to run the program, the random module to create random locations for the food, and the FreeGames library, which makes it easy to create simple and fun games with graphics. Importing these libraries is necessary because without them, the program would not be able to run. We can import these libraries using this code:
Next, we need to set up some of the main variables for the program. We will do this by creating a variable called food, then setting it to the vector (0, 0). This will set the first piece of food’s location to the very center of the window. Next, we’ll create a snake variable, but the vector will be set to (10, 0). Finally, the snake would be facing down, so we will create a variable called “aim” and set the vector to (0, -10). Once this step is complete, the code will look like this:
Step 2: Create the Change and Inside Functions
Now it’s time to create the function that will allow us to change direction and check whether the head is inside the main screen. For the change function, we will type:
What this function does is it simply takes the x and y coordinates for the aim variable, and adjusts them to fit the new value. This will allow the user to turn the snake and make it change directions. The inside function is also a very simple function. It involves taking a parameter of the snake’s “head” (the leading block), and checking if it within -200 and 190 in both the x-and y-directions. Once this code is complete, it should look like this:

Step 3: Create the Move Function
Next we need to create the main function that will move the player’s snake. Start by defining the function, and we’ll set the head equal to the start of the snake, then make it move in the direction of the aim variable. Then, we’ll check if the head is within the boundary of the screen or touching the rest of the snake. If so, we will draw a red square where the head is and update the game to end it. We will return this value, then use an append command to add it to the snake. The code for this section of the function will look like this:
Then, we need to check if the head’s location is equal to that of the food. If so, we will print “Snake:'' and the number of points that the player has. We will then use the randrange method from the random library to generate random X and Y-coordinates for the food to appear at next. However, if the head is not touching the food, the last value in the snake will be returned and removed in order for it to look like the snake is moving. Finally, we’ll clear the screen in order to keep the game up-to-date. Once this is done, the code will be:
Finally, we just need to set up the snake’s body and then the move function will be complete. To do this we will type:
What this code does is begin by setting the color for each of the snake’s squares to black. Then, the food color is set to green, in order to make it easy to see and discern from the snake. Then, the game updates and the time is reset in order to prevent glitches. And with that, this step of the program is complete!
Step 4: Finish Program Setup
Finally, we’re on to the last step, which will include setting up the window, as well as creating some small functions that will allow the player to control the snake using the keyboard. We’ll start by setting up the window, by entering the coordinates (in this case, we’re using 420, 420, 370, 0). Then, we will hide the turtle cursor as well as the tracer, which will make sure the turtle doesn’t leave a line behind it. Then, we will call the listen() command, which will make the program listen for user input. The next step will involve creating four lambda functions to control each of the computer’s arrow keys. A lambda function is a function that can be created without a name, which makes it perfect for this purpose. Each arrow key will change the turtle’s direction by 10 pixels in the given direction. Finally, we need to call the move function and add in a done() command and the program is finished! Once all of this is complete, the code will look like this:

Project Complete!
Now the project is complete! We hope you’ve enjoyed this simple Python project to create a fun and simple snake game, which can be played either with friends or alone!

Feel free to test your code now and see how it works. If you’re stuck or have any issues with your code, try reviewing it again either in your text editor or by looking at the code included in the article as a reference.