Introduction:
In this article, we are going to be using Python to create a simple desktop notifier program. This program will allow users to set and schedule reminders to appear on their desktops at any time, making it easy to stay on track and motivated to complete tasks.

This project is a great way to learn more about making functional programs by creating a program with a variety of uses. It’s a great way to build both your creative thinking and problem-solving skills while making a functional computer program.
Who is this Project For?
This is a beginner-level project for those who are new to Python, or those who want a fun way to apply their coding skills. Before starting this project, you should have some experience using if-statements and taking user input. These are two simple concepts but will be used and built upon as you code this project.
What Will We Learn?
This program focuses on creating a functional reminder program to deliver notifications to users’ desktops. This teaches concepts such as if-statements, taking user input, and using libraries such as the time and plyer libraries.
Features to Consider:
- The user will be able to select a title for their reminder
- Users will be able to customize the message that will be displayed on their notification
- There will be the option to enter a scheduled time (in minutes) to display the reminder
- If the user does not select a time, the reminder will be displayed right away
Pseudo Code:
Below is the pseudocode for this game:
<code>
Import the time library
Import the notification module from the plyer library
Display the message “------ Add A Reminder: ------"
Print the program description- "Welcome to the reminder program. Below you will be asked to enter a title and message for your reminder."
Ask the user what they want to title their reminder
Store the title as a variable
Ask the user what their reminder should say
Store the message as a variable
Ask the user if they would like to schedule the reminder (this will be a yes or no question)
If yes:
Ask the user how long from now they want the notification to show up
Store this value as a float
Wait for as many minutes as the user specified
Display the notification
Else if no:
Display the notification
Else:
Print “Sorry, that is not an option. Please try again.”
</code>
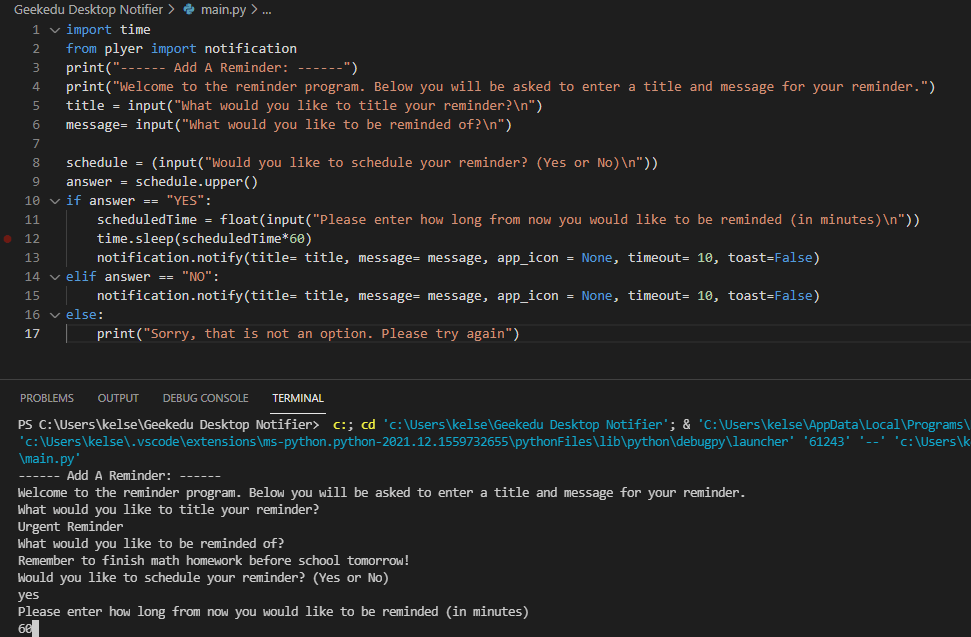
Main Steps:
This project can be broken down into 3 main steps:
- Import libraries
- Take user input
- Schedule the reminder
Step 1: Import Libraries
The first thing we’ll need to do when creating this game is import all of the required libraries. Libraries are essentially a set of pre-written functions, and that make coding much easier. For this program, we will be using two libraries: time and plyer. The time library will be used to create a time delay between the current time and the time the user wants their reminder to appear. The plyer library will allow us to create the notification to be displayed on the desktop. We can import these libraries using this code:

Step 2: Take User Input
Next, we need to add some text to explain what the program is and how it works. In this example, we are adding a title as well as a short two-sentence description to let users know what to expect. The code for this is as follows:
Now it’s time to ask the user to enter the title and the body text for their reminder. We will use an input statement to ask the question and assign the user’s response to a variable. To complete this step, we will type:
Finally, we just need to ask the user if they would like to schedule their reminder to notify them at a different time. To do this, we will start by taking input as we did before, but we will use the float() method to make sure that the input is taken as a float (or decimal number). We will also use the upper() method to convert the user’s input into all uppercase letters, which will make it much easier for the program to respond appropriately based on the user’s choice. Once this code is complete, it should look like this:
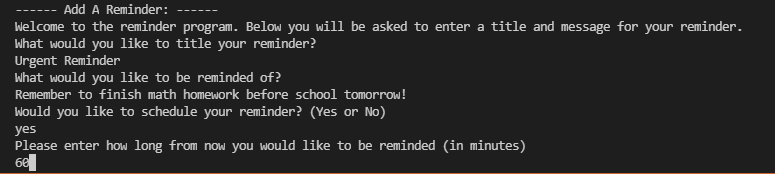
Step 3: Schedule the reminder
Finally, we need to schedule the reminder to be delivered. We’ll start by creating an if-statement to handle what happens if the user wants their reminder to be scheduled. In this case, we will ask them how long from the current time (in minutes) they would like to be reminded. Next, we will multiply this number by 60 to get the number of seconds that this will be. The python time.sleep() method only takes input in seconds, so this step is essential! Finally, we will display the notification for the user. The code for this section will look like this:
Hint: As you can see, the notification.notify() method takes parameters such as the title, message, icon, and timeout. These can all be customized depending on your preferences, so feel free to test them out and make this project your own!
Finally, we just need to add the rest of the if-statement. If the answer is NO, then the reminder will be displayed right away, and if the answer is neither yes nor no, the program will simply say to try again. This is the final section of code for this project, and it is as follows:
Project Complete!
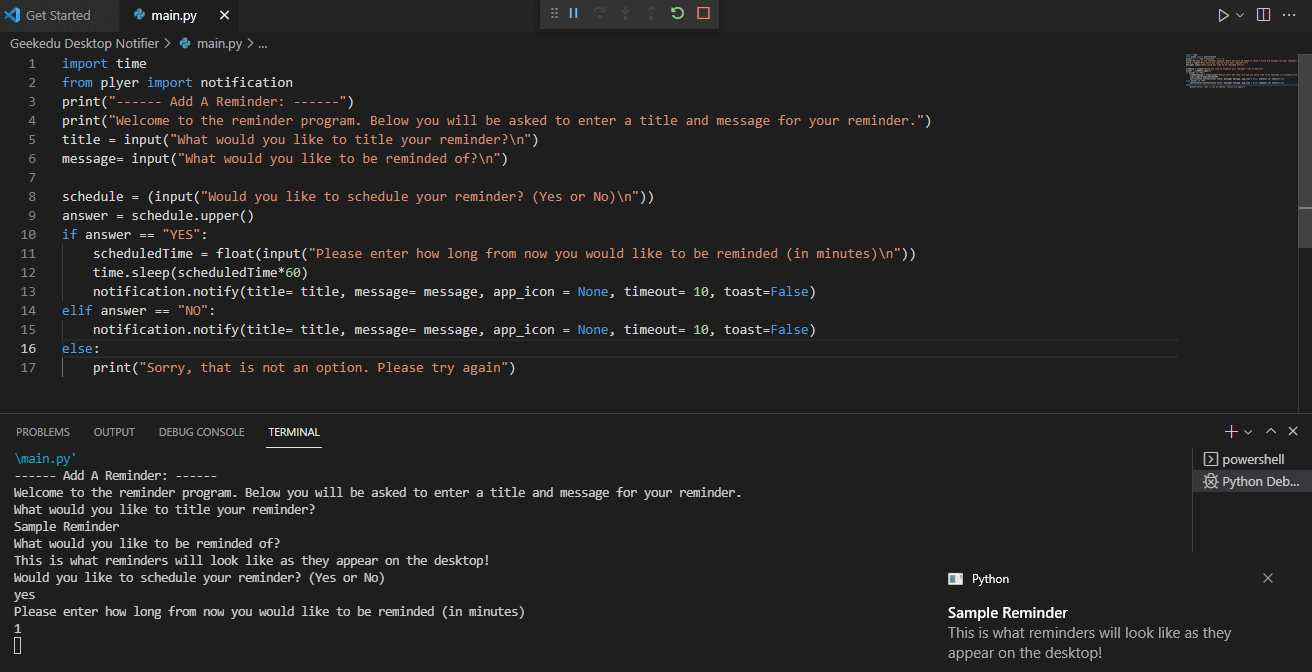
Now the project is complete! We hope that you’ve enjoyed creating this desktop notifier application. At this point, it should be fully functional and you will be able to use it to remind yourself to work on tasks and to help keep yourself organized. Feel free to test your code out and if you have any errors, make sure to check the code mentioned above as a reference.