What is the project?
In this project, we will be using Java to create an interactive menu application for the user. The objective of the project is to prompt the user will a list of options and then give them a their total price
How does the project work?
When the program runs, the user will be greeted by the application and will then be prompted a list of foods to choose from (the prices and food choices will be chosen by the students). Once the program is finished, prompt the user with the menu it will then prompt the total price.
Who is this for?
Java Experience: Beginner
Challenge Level: Beginner
Project Goals
In this project, students will have the opportunity to create and design their own interactive menu. This project is perfect for students who want to get familiar with formulas and basic math in Computer Science. The logic they are learning will help them build the fundamentals for more complex mathematical problems in the future
Code To-Do List:
Materials and Resources
Materials
Code Editor: Replit IDE (Integrated Development Environment)
Start Coding Here: https://replit.com/languages/java10
Resources
In this project we will be using a couple Java libraries to bring this project alive.
Java Libraries being used:
java.util.Scanner
java.text.NumberFormat
Implementation
Step 1: Let’s organize our code by placing comments
Why do we comment code?
As developers, it is very important to place comments into your code because it makes your code more readable to other programmers. This way when a problem occurs within your code it can be easily spotted because your work is already annotated and there is a description on how each function and/or statement is doing.
- Before we actually start programming let’s title our work.
At the first line in our program, let’s add our Name, Date, and Project Title by using Java comments.
- Now, let’s section our code into comments to make it organized. There will be three different sections. The first section will be for all the libraries we imported and are using. The second section we will be adding our prices. The third section we will be adding our prompts. The fourth section we will be adding our formula and prompting the user for the final total.
Step 2: Importing the Java library we will be using
Why do we use libraries in Java?
One of the reasons Java is such a great language to program in is because of the vast variety of Java libraries it offers. Using these great libraries cut off time writing code from scratch because other developers were kind of to make the program libraries so it would be a lot easier for us to make fun cool projects like the Fun Menu Project.
- Now, Let’s import all the python libraries.
Step 3: Now let’s get into the fun stuff
- First, let's choose our prices and foods. The variables we will be using final doubles. Final means that the value of the variable can not be changed within the class. This will be great when we are making our food variables.
- Now we have the prices, let’s declare our java scanner.
- Great! We have the scanner ready let’s begin prompting the user
Let’s type:
- Once we have our questions ready we can now create our formula. We need to create a variable for the formula. So, the variable will be a double and the double value will consist of Burger * numBurgers + Fries * numFries + Softdrink * numSoftdrink. This value will equal their total cost of their order. The number format will give the user the total in dollar value format.
Output of the project:
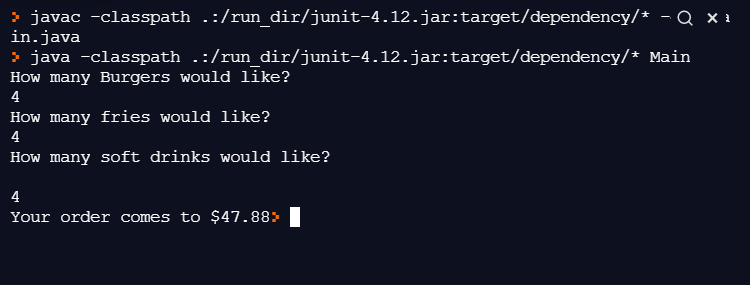
Congratulations, you have completed the project!