Introduction:
In this article, we are going to be using Python and the Tkinter library to create a simple paint program that will allow you to create artistic masterpieces. The program will include a pen, a color selector, a menu to choose a pen size, and an eraser.

This project is a great way to learn more about making functional programs by creating a useful program that can be used for a wide variety of purposes. It’s a great way to build both your creative thinking and problem-solving skills while making a functional computer program.
Who is this Project For?
This is an intermediate project for those who are new to Python. Before starting this project, you should already have experience with classes, using the __init__() method, and creating simple Tkinter GUIs (Graphical User Interfaces) as these are the concepts that you will use and build upon as you code this project.
What Will We Learn?
This program focuses on creating a GUI using the Tkinter library, which is used to display the print program. Classes and methods are also used to set up the ‘self’ object and to create the program’s functions. These concepts are more advanced but are important to understand, making this the perfect program to improve these essential skills.
Features to Consider:
- Users will be able to choose a pen size and color to draw with
- The pen will follow along with wherever the user’s mouse moves
- The eraser tool can be used to erase sections of a drawing
Pseudo Code:
There are many ways that this game could be programmed, but below is the pseudocode that was used to make this paint program:
Import Tkinter and colorchooser libraries
Create an object called paint, with the color black and a size of 10 pixels
Create the Tkinter window
In row 0, column 0, create a button called “pen” that runs the function usePen
In row 0, column 2, create a button called “color” that runs the function chooseColor
In row 0, column 3, create a button called “eraser” that runs the function useEraser
Orient the button horizontally
Create a “choose size” button in row 0, column 4
Create a 600x600 canvas with a white background
Set the canvas to row 1 and ake it span 5 columns
Run the setup function
Run the mainloop function
Function setup(self):
Create variables for old x and y-coordinates and set them to 0
Set the default line width to 0
Set the color to the default color
Set the eraser state to off
Set the pen button to active
Function usePen(self):
Use the pen if the pen button is active
Function chooseColor(self):
Set the eraser to false
Set the color to the user’s chosen color
Function useEraser(self):
Set eraser to true
def buttonPressed(self):
If the button is pressed style it to look sunken in
If the button is not pressed return it to its’ original state
Function paint(self, event):
Set the pen size to the user’s selected size
If the eraser is on, tet pen color to white, otherwise, set it to the selected color
Draw a line from the old x and y-coordinates to the pen’s current location
Set the old x and y-coordinates equal to the current x and y-coordinates
Run the paint function
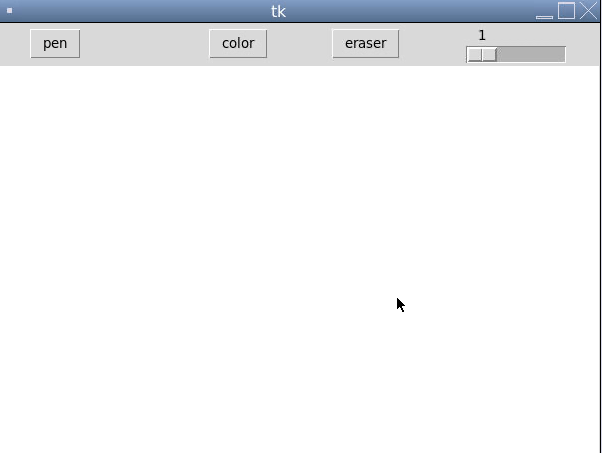
Python Paint Program
Main Steps:
This project can be broken down into 4 main steps:
- Import libraries and set up the Paint class
- Create the Self and Setup functions
- Create the Pen, Erase, Colour, and Size functions
- Create the Paint function
Step 1: Import Libraries and Set Up The Paint Class
The first thing we’ll need to do when creating this game is import all of the required libraries. For this program, we will be using the Tkinter library to create the GUI as well as the askcolor module to allow users to select multiple colors for their pen. Importing these libraries is necessary because the program would not be able to run without them. We can import these libraries using this code:
Next, we need to set up the Paint class. This is a class that will store objects used in the program, and it is what will be called in order to make the program function. This will be done using the general class definition syntax, as seen below:
Hint: You will also notice that as a part of the class we have included two variables. These will be used to set default pen colors and sizes for the program, so feel free to change them to whatever you’d like.
Step 2: Create the Self and Setup Functions
Now it’s time to create Self and Setup functions for the program. The self function will simply set up the main GUI window, which includes buttons, the canvas, and the menu to choose a pen size. The setup function will set up the pen by setting its coordinates, color, size, etc. However, we will start with the self function so that it can be used later in the setup function. For the self function, we will type:
Note that the majority of this function consists of creating buttons and aligning them in the GUI. Each of these buttons follows the same format as well - the command takes input for the button text, function, and alignment using columns and rows. The general format is as follows:
Now that the self function is complete, it’s time to create the setup function. This function’s code should look like this:
The first couple lines of code ensure that no pen coordinates are already stored in the program. Then, we will add a line of code to check what pen size and color the player has selected and these are applied to the pen. Finally, we will set the eraser to false and then check the status of the pen button to make sure that it has been selected. Once this is done, it’s time to move on to the program’s other functions

Step 3: Create the Pen, Erase, Colour, and Size Functions
Now that we have finished creating the self and setup functions for this program, it’s time to secret the rest of the functions. We will start by creating the pen and color functions. These functions are both fairly short and simple, as they both simply take input from the button or selection menu that they are associated with. The code for this section will look like this:

Python Paint Program
As you can see, the usePen function simply checks the state of the pen button, while the chooseColour function sets the eraser to false and sets the pen to the color that the user has selected. Next, it’s time to create the useEraser and buttonPressed functions. The eraser function is almost the same as the usePen function, except it sets the state of the eraser boolean to true. The buttonPressed function, however, is a little different. It involves formatting the button to change looks when it is actively being pressed, as well as changing the setting of the eraser-mode variable to turn the eraser on. Once this is complete, the code should be:
Step 4: Create the Paint Function
Finally, we’re on to the last step, which will include creating and calling the paint function so that the game will run.
Note that each of these labels follows the same format: the text that will be written, the font and font size, then the color of the text. This format will be used for each label, meaning that once you make one it is simple to make the others using the same format. Finally, we will create an input box where players can type their response, and a start button to begin the game, and then the game is done!
Once all of this is complete, the code will look like this:
As you can see from the code above, the first step is to set the line width to whatever the selected size is. Next, the paint color is set to white when the eraser is on. Next, there will be a line drawn from the old point to the new point in the canvas, and the mouse’s location is followed to ensure that the pen draws on the canvas. Once this section of code is finished, the project is done!
Project Complete!
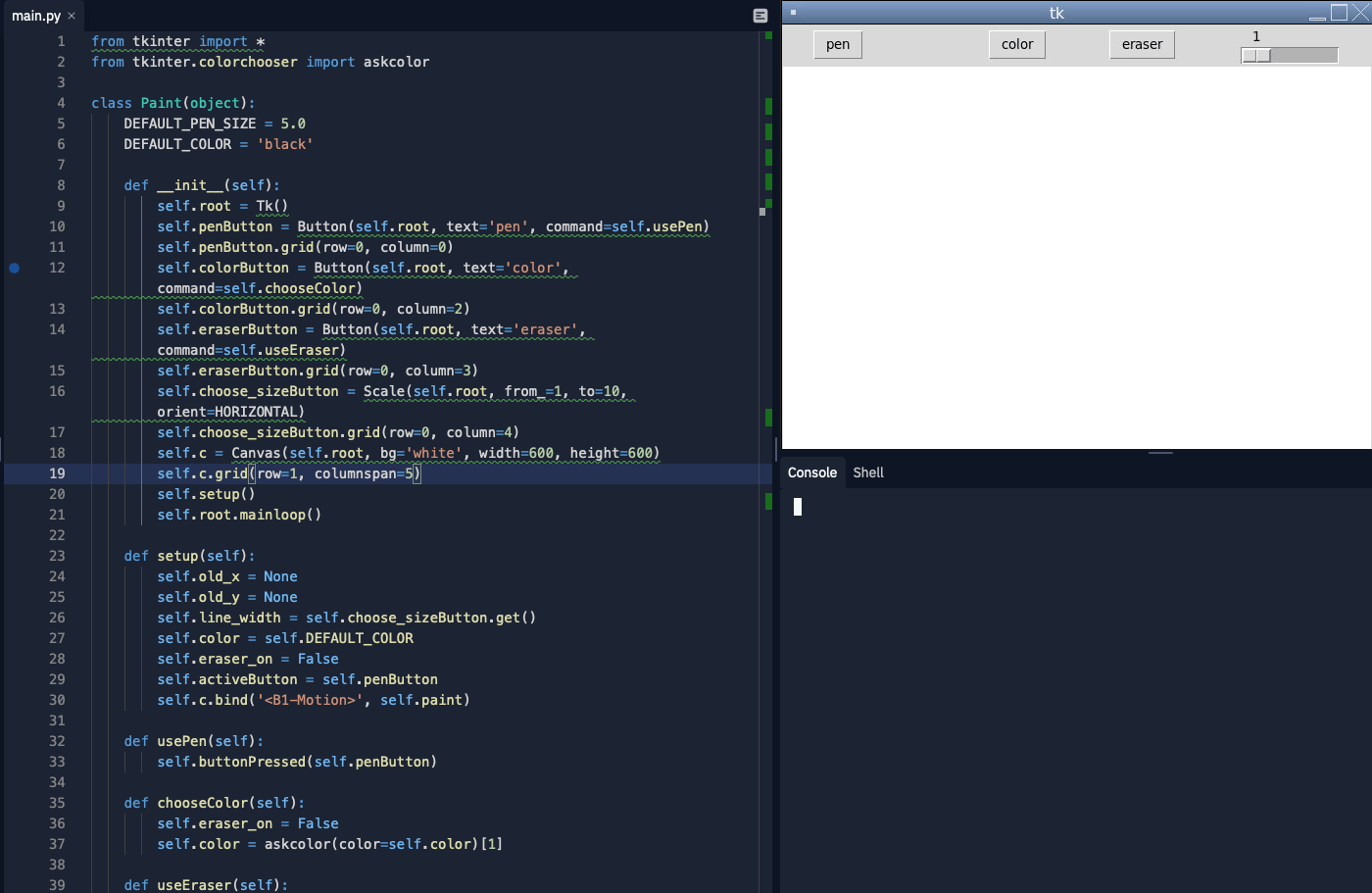
Now the project is complete! We hope you’ve had fun creating this paint program using Python and the Tkinter library, and hopefully you’ve learned more about programming with Python! Feel free to test your code now and see how it works. If you’re stuck or have any issues with your code, try reviewing it again either in your text editor or by looking at the code included in the article as a reference.