Introduction:
In this article, we are going to be using Python to create a simple typing test program. This program will ask users to type a sentence and will let them know how many errors they made and how fast they typed the phrase.

This project is a great way to learn more about making functional programs by creating a functional and versatile program. It’s a great way to build both your creative thinking and problem-solving skills while making a useful and practical project.
Who is this Project For?
This is an intermediate-level project for those who are new to Python, or those who want a fun way to apply their coding skills. Before starting this project, you should have some experience with creating and using functions, loops, and conditional statements such as if-statements. These concepts are widely used in any computer program, and your knowledge of these concepts will be developed as you work through this project.
What Will We Learn?
This program focuses on creating a functional reminder program to deliver notifications to users’ desktops. This teaches concepts such as if-statements, functions, and using operators as well as other essential programming concepts.
Features to Consider:
- The user will be given a phrase to type
- They will press enter to begin the timer, type their sentence, and press enter to end the timer
- The program will check how many errors the user made and will present them with their time and accuracy results.
Pseudo Code:
The pseudocode for this project is included below:
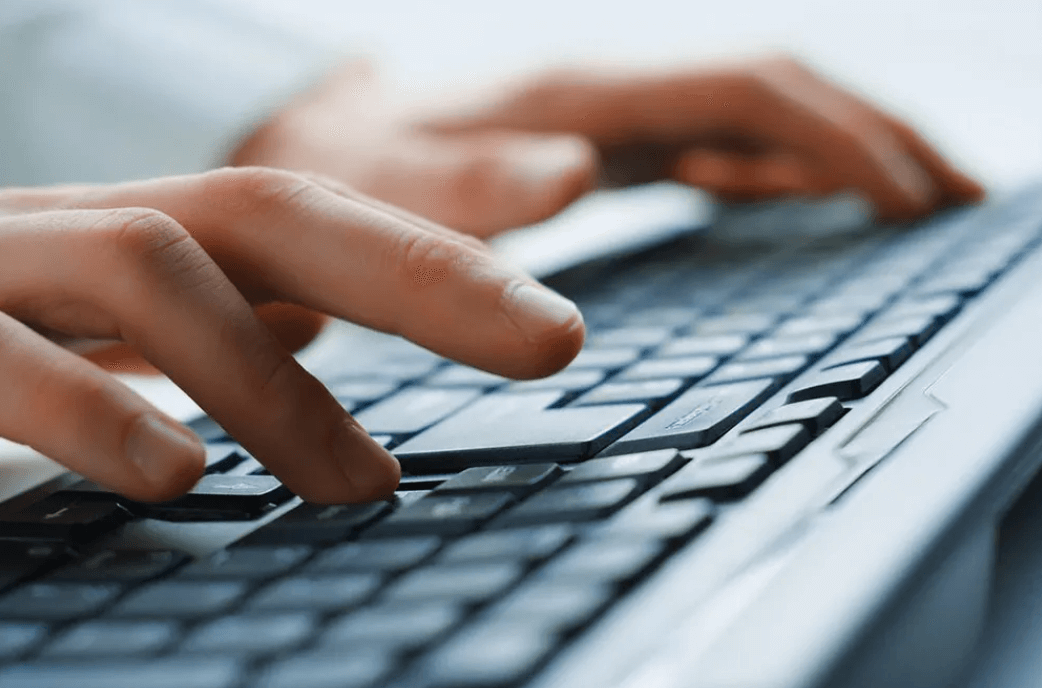
Main Steps:
This project can be broken down into 3 main steps:
- Create the typingErrors function
- Create the typingSpeed and timeElapsed functions
- Create the program’s input and output
Step 1: Create the typing Errors Function
The first thing we’ll need to do when creating this program is to import the time library. This will allow the program to time how long the user takes to enter the sentence in the program and will let them know how fast they can type. Then, we’ll need to create our typingErrors function to check how many errors the user makes while typing.
As you can see, this function breaks the long sentence down into smaller words and checks whether the user has typed them correctly. If not, their error score is incremented by 1.

Step 2: Create the typingSpeed and timeElapsed Functions
Next, we need to create the typingSpeed function. This will be used to calculate how many words the user types per minute. The code for this function is as follows:
Now it’s time to create the timeElapsed function to check how long it takes for the user to type their sentence. To complete this step, we will type:
As you can see, we now have two functions that can be used to calculate the user’s score by telling them their time and how many words they can type per minute.
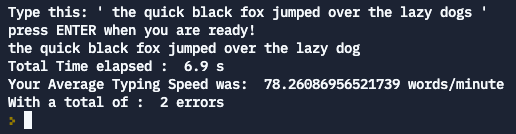
Step 3: Create the Program’s Input and Output
Finally, we need to create the program's input and output and set up some of our variables. We will begin by creating a prompt that the user needs to type in, and then printing it and asking the user to press the enter key to begin the timer. Then, we will use the time library to select the correct values for stime, etime, time, and speed. Finally, we will print the user’s results and the program is done! This is the final section of code for this project, and it is as follows:
Project Complete!
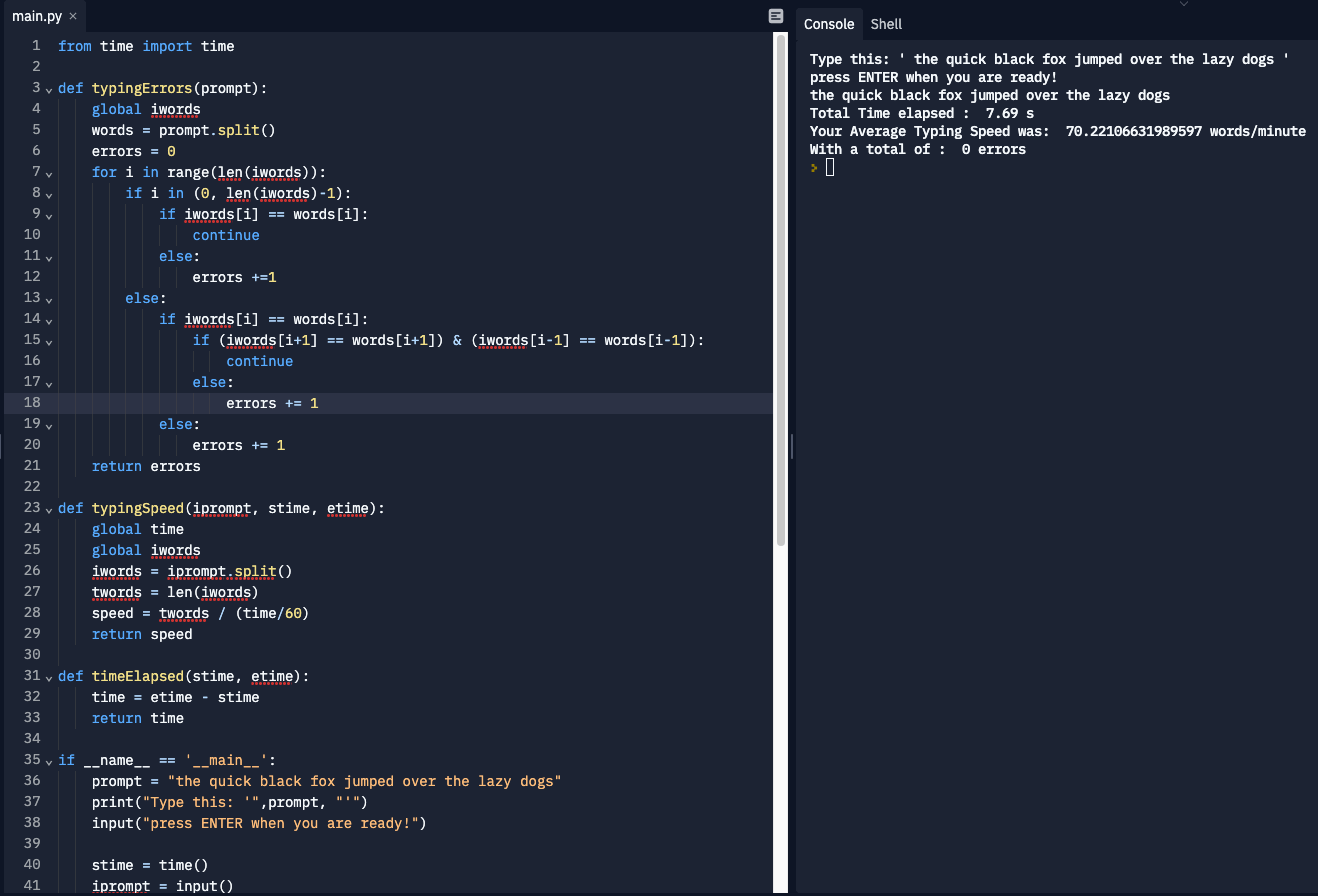
Now the project is complete! We hope that you’ve enjoyed creating this simple typing test. Feel free to test your code out and check the code mentioned above as a reference if you have any errors.