In this tutorial, we are going to look at an explained solution of the problems in the Programming contest (Senior Category) on Canadian Computing Competition 2022. The solutions and explanations are made by keeping in mind that this is your first participation in a programming contest. I would suggest you try to code first after reading the explanation and before seeing the solution code. Keep practicing and solving the problems. Happy Coding!
Problem S1: Good Fours and Good Fives Statement:
You are given a number. You have to print the number of ways, this number can be represented using a sum of fours and fives. Number of fours or fives must be different in each way.
Idea:
If we take k number of fives, can you say whether we can make sum using any number of fours? We can make desire sum if the remaining value (that is n-k*5) is divisible by 4. So, one optimal way is to run a loop from 0 to n, and check for every possible number of fives, if it’s possible to make sum equal to n just increment your answer by 1.
Complexity: O(n) here n can be upto 1e6. As we are iterating from 0 to n;
Flowchart:
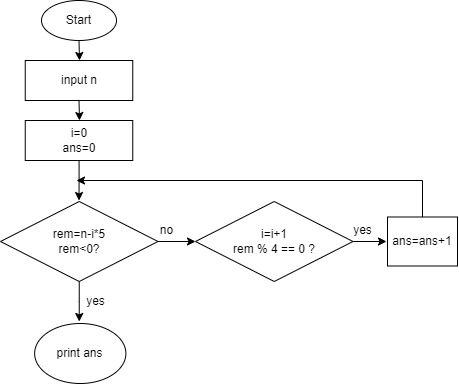
Code: C++

Problem S2: Good Groups Statement:
You are given some number of groups. Each group consists of three members. There are two types of constraints:
- given two names, they must be in the same group
- given two names, they must be in the different group.
Now you have to count number of groups that violate one of these two constraints.
Idea:
For each type (i) and type (ii) constraints, you have to check whether they are in the same group or not. How can you determine the two members are in same group? We can use a disjoint set union to check it. Disjoint set join stores the connected components and all these connected components have the same parent.
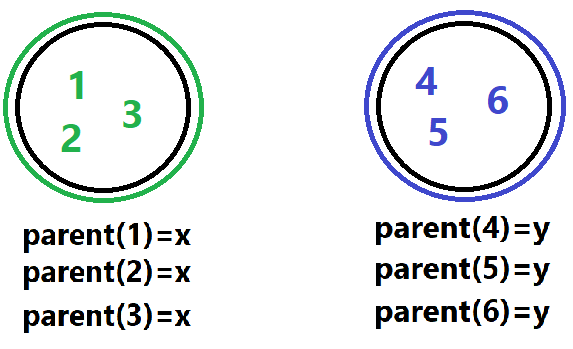
So for each two members, if their parents are same for type (ii) constraint that means this constraint is violated. We will increment our answer by 1. Can you do the same for type (i)? I think so. Now, the important part is to store the names of each member. One possible way is to store the hash value of each name. Instead of using hash value, we can use STL map, that takes log(n) time to store a name and it’s fine as we have enough time to execute all test cases.
Complexity: O(nlogn) as there are n names, each takes logn time to store.
Flowchart:
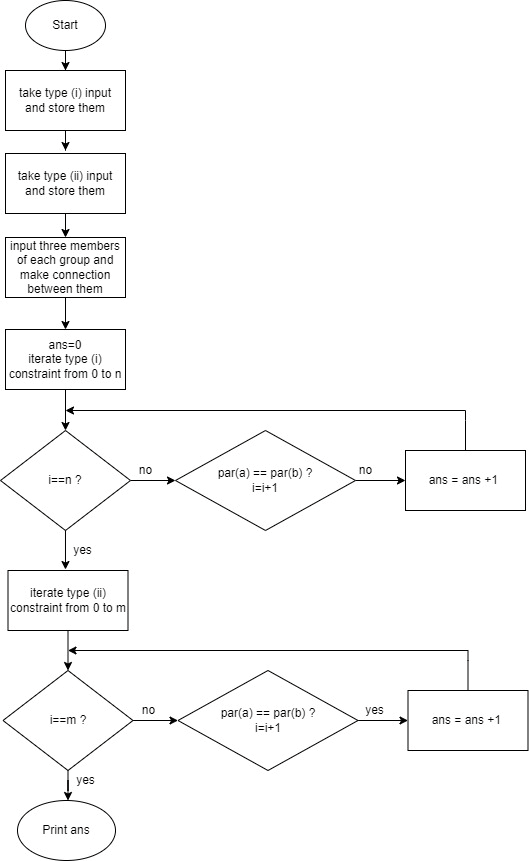
Code: C++

Problem S3: Good Samples Statement:
You are given a series of notes. Each note is an integer. You can select any non-empty sequence of consecutive notes without any repetition that means all notes in a selected sequence must be distinct. This selected sequence is called sample. You have to construct a series of notes of length n where each note will be between 1 to m (inclusive) and total number of sample will be exactly k. can you do this?
Idea:
Let’s consider this sequence:
5
1
2
Each element in this sequence is distinct. How many new samples will be created if I add a distinct number at 4th index of this sequence?
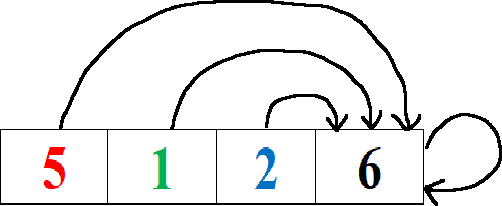
Here we can see, 4 new samples will be created. They are [5,1,2,6], [1,2,6], [2,6], [6]. So, If I want to add another distinct number at 5th index, another 5 new samples will be created. But if I add a number that is not distinct, how many new samples will be added? Let’s see the below figure:

Only 3 samples are added. They are [2,6,1],[6,1],[1]. So, here’s the conclusion:
If we add a distinct number at position k, total k new samples will be added.
If we add a non-distinct number at position k, total (k-previous position) new samples will be added. To construct a series of n numbers,
We can run a loop from 0 to n-1. To find a number for the i th index, we will make a decision whether we will select a distinct or non-distinct number by observing the remaining value of
k. After selecting number, store it to a container and total number of new samples will be subtracted from k. Outside the loop, we can simply check the value of k. if value of k is zero, that means, we have successfully created our desired series. Just print all stored values. Otherwise print -1.
Complexity: O(n) where n is the length of series.
Code: C++

Problem S4: Good Triplets Statement:
A circle is given with (0,0) center. The circumference of the circle is C. So there can be total C integer points on the boundary of the circle. Total n points are drawn. The location of the points on the circle is the counter-clockwise arc length from the right-most point of the circle. Multiple points can be drawn at the same location. Your task is to calculate the number of ways to select three points in such a way that if we create a triangle using this three points, circle’s center will be strictly inside the triangle.
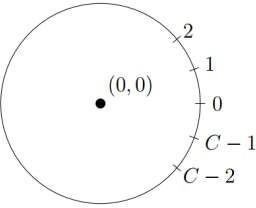
Idea:
We will follow a different approach to solve this. How many ways are there to select 3 points from n?
It’s = n*(n-1)*(n-2)/6. But we can’t include all these ways to our answer as some ways won’t make a triangle that satisfy conditions. We must subtract the number of ways to select 3 points from n that don’t satisfy conditions. So,

ans = n*(n-1)*(n-2)/6 – (number of ways that don’t meet the conditions)
Now, our target is to calculate number of ways of select 3 points from n that don’t meet the conditions. We will iterate every points that are drawn on the circle and count how many ways are there to select another two points such that these points create a triangle that don’t meet the condition. We sum up all these ways and subtract it from

. How can we do this?
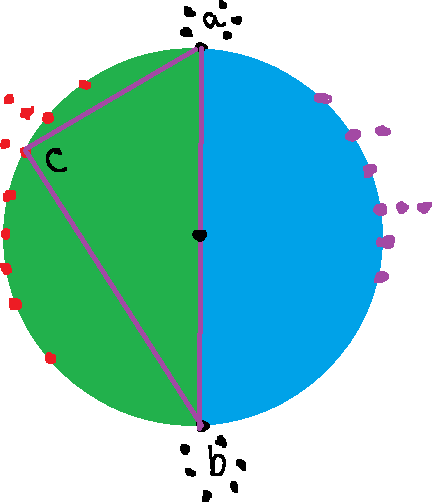

Say, we are on point a, we will find the opposite point of a and it’s b. if the circumference is C, then opposite point of a will be (a+c/2)%c. Look at the above right sided figure, the circle’s circumference is 8, so opposite point of 2 is (2+8/2)%8 =6 . For a particular point a, we will find opposite point of it (b). Calculate total number of points from a to b in counter clock-wise. we are selecting these because using any three points from these, creating triangle won’t meet
the condition as their center won’t inside the triangle. For better understanding, look at the left sided figure. There can be some cases to find all the ways from these points:
- select a single point from a, take another two points from a to b
If number of total points at a is Ca, and number of total points from a to b is Cb then total ways = Ca * (Cb-1)*Cb/2 by combinatorics
- select two points from a and take another one point from a to b
Total ways = Ca * (Ca-1)*Cb/2
- select all three points from a
Total ways= Ca * (Ca-1)*(Ca-2)/6

Similarly, when we are on b, its opposite point will be a. then calculate total number of points from b to a in counter clock-wise and follow the same manner when we calculated the total ways for a to b. After summing up all these ways, we will subtract this value.
Critical observation: when C is even, and we calculate ways from a to b, and ways from b to a. points that are on a and b are calculated twice.
Complexity: O(n) where n is the circumference of the circle.
Code: C++
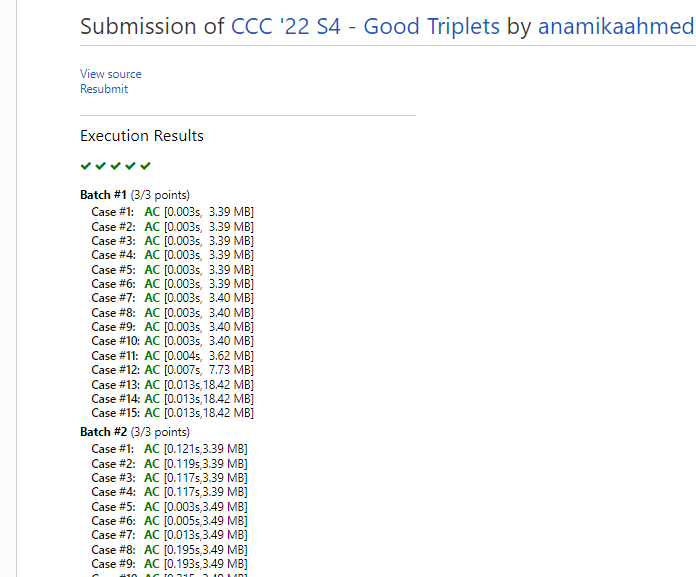
Problem S5: Good Influencers Statement:
You are given a tree of n nodes. Each node represents a student. If a student intends to write CCC, then it’s Pi will be Y, otherwise N. You can select any node with Pi=Y and pay costi dollars to force all its neighbors to write CCC. You can repeatedly select node with Pi=Y. You have to intend all students to write CCC with minimum total cost.
Idea:
Let’s introduce dynamic programming first. DP is a technique that is used in a situation where you have to calculate the same value in multiple times. Instead of calculating same value again and again, we can use DP that will store value in that state, we can simply return DP value. It minimizes our overall time complexity.
A student can act as following three:
- it intends CCC already, minimum cost is denoted as DP[i][0]
- it will be influenced by its parent, minimum cost is denoted as DP[i][1]
- it will influence it’s parent, minimum cost is DP[i][2]
Now, select a root arbitrarily, say it’s 1. We will traverse the whole tree. When we are on node i, we need to calculate the minimum cost required to solve the subtree of node i. So, calculate DP[i][0], DP[i][1],DP[i][2]. Let’s observe the following cases:
- if Pi=Y or state=1
It denotes i th node is intended directly or indirectly. Now we can pay cost to this node to influence it’s child neighbor node. We have two options here. We can pay or not to pay. Take the minimum value.
- if Pi=N or state=0 or state=2
It denotes i th node is not intended yet. So, we have to pay at least one of its child to influence its parent node. Which child should we select? at first, we will sum up the cost of all child subtree. If we have several child’s like x1,x2,x3. Calculate the minimum cost for all these child, and take minimum value to influence our parent node as it is not intended. If it is not possible to active the whole tree without paying parent node, just return INT_MAX. Maintain the all these critical cases separately. If you stuck, following code section can be helpful.
Complexity: O(n) where n is the total number of nodes
Code: C++
