Introduction:
In this article, we are going to be using Python and its’ Turtle and Freegames library to create a fun Tron Game. In this game, two players control lines and move them around the screen. The goal of the game is to get the other player to collide with your line before you collide with theirs.
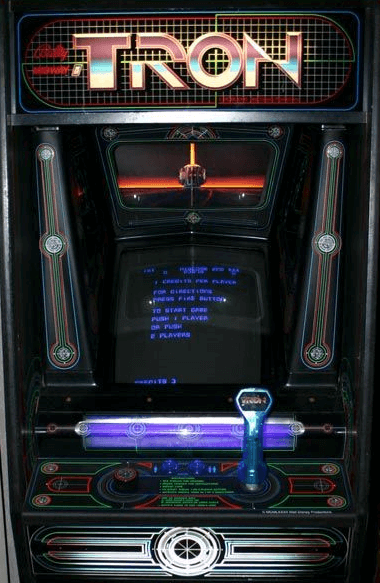
This game is both fun to make and simple to play. Making it the perfect project for those who are looking for a way to improve their coding skills and make a project.
Who is this Project For?
This is a beginner-level project for those who are new to Python. Before starting this project, you should already have experience with using operators and booleans, creating functions, and using if-statements, as these concepts will be used throughout the project.
What Will We Learn?
This program focuses on using operators and functions to control gameplay and what happens when the player performs any actions. The use of coordinates and vectors are also important as they are used to control the players’ directions and placements. These concepts are common to many coding-related projects, and completing this project will help you gain a deeper understanding of how they are used.
Features to Consider:
- Users will use the A&D and J and L keys to control their players
- Each time a key is pressed, the player will rotate 90 degrees in that direction
- If a player bumps into their opponent or the edge of the game screen the game ends
Pseudo Code:
Here is the pseudocode for this Tron game:
Main Steps:
This project can be broken down into 3 main steps:
- Import libraries and set up the main variables
- Create the game’s functions
- Finish game setup
Step 1: Import Libraries and Set Up The Main Variables
The first thing we’ll need to do when creating this game is import all of the required libraries. For this program, we will be using the Turtle library to make the game screen, and the Freegames library to handle some game actions, such as the vectors and squares that are used later in the code for this project. We can import these libraries using this code:
Next, we need to set up the main variables for the game. We will begin by creating variables to store each player’s coordinates, direction, and a variable to represent each player’s ‘body’. Once this has been done, the code is:
Step 2: Create the Game’s Functions
Now that we have finished creating the variables needed for this project, it’s time to begin coding the game’s two main functions. The first function will be called ‘inside’ and will check whether a player’s head is inside the game screen. This is important because if the head is no longer inside the game screen, the game will end and that player has lost.
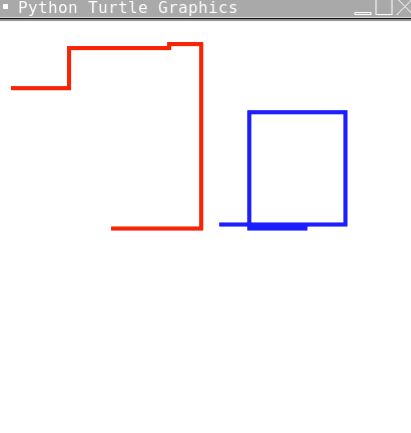
The second function will be called ‘draw’ and will draw and set up the game screen and simple graphics. The code for this function is as follows:
Now that the first three functions are complete, we have two more functions to create. We will create a function called tap which will control the detection of clicking on any of the tiles, as well as what happens when each tile is pressed. We will also create a function called draw, which will combine some of the code we wrote previously to draw the grid on the game screen. The code for this step will look like this:
As you can see, the code above handles players colliding with one another, controlling the initial aim of each player, and gradually increasing the length of each player’s line. Now that these functions have been created, it’s time to add the final lines of code that will call these functions and run the game. At this point, the game code is mostly functional, but we have not called any of these functions so they will not run.
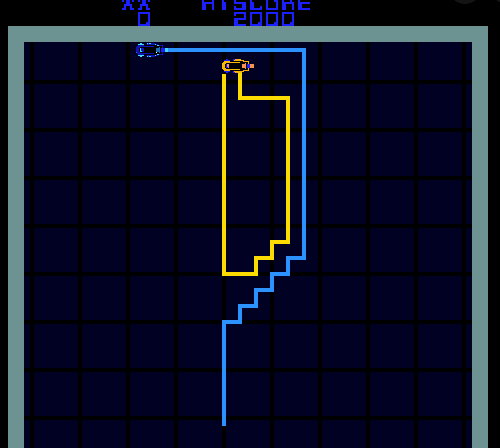
Step 3: Finish Game Setup
Now that all of the game’s functions are done, we need to set up the game screen, add a couple lines of code to call these functions, and create the lambda functions that are used to control which way each player moves. The code for this final step is seen below:

Project Complete!
Now the project is complete! We hope you’ve had fun creating this fun Tron game, and hopefully, you’ve learned more about programming with Python! Make sure to test your code now and see how it works. If you’re stuck or have any issues with your code, try reviewing it again either in your text editor or by looking at the code included in the article as a reference.