Introduction:
Many people love listening to music, whether it be classical, country, rap, pop, or any other genre. Music is a great way to connect with others and get to know those with similar tastes or can be used to broaden your interests through listening to different music. However, sometimes it can be annoying when you just can’t seem to remember or understand the lyrics to a song that you just listened to.

That’s why in this article we are going to be using Python to create a song lyric extractor that will take user input and display the lyrics to any song the user inputs. Whether you are new to Python or have some prior experience, this project is a great way to learn more about making functional programs and helps you apply your knowledge in a practical and fun way. Simple and fun projects like this make great beginner Python projects for kids.
Who is this Project For?
This is an intermediate-level project for those who are new to Python. At this point, you should already know the basics of creating functions and creating simple GUIs (graphical user interfaces) with Tkinter, as well as using APIs (Application Program Interface) to gather information from other websites. These are the concepts that you will use and build upon as you code this project.
What Will We Learn?
This song lyric extractor project focuses on using the Tkinter library to create the program’s GUI, as well as the lyrics_extractor module to get song lyrics from an online source and display them in the GUI. Throughout the project, you will learn about creating functions, labels, and buttons, as well as how to use basic APIs to make a functional project.
Features to Consider:
- The player can enter any song name into the input box
- When they press the “show lyrics” button, the lyrics are shown in the GUI
- The program will display accurate song lyrics based on the inputted song title

Main Steps:
This project can be broken down into 4 main steps:
- Import and setup modules
- Create the main Tkinter GUI
- Define the “Get Lyrics” function
- Create the GUI’s ‘Show Lyrics’ button
Step 1: Import the Modules:
The first thing we’ll need to do is import all of the libraries and modules that we’ll be using for the project. Because the Tkinter library is non-native, it must be installed and imported to the computer before being used. The first step we will need to do is import the Tkinter library and the lyrics_extractor module into the program. This will let the program know that we will be using them. The code for this step will look like this:
Note that in the code above we imported specific objects for each module. For Tkinter, we used the asterisk (*) to import all Tkinter objects, but for lyrics_extractor, we only imported SongLyrics.
Step 2: Create the main Tkinter GUI
Now that all of the modules have been imported, it’s time to create the program’s graphical user interface. This will involve setting up the canvas and its dimensions, titling the window, and adding a text input box. To begin, we’ll initialize Tkinter and create the window. Then, we’ll title the window so that users know exactly what it’s called. In this case, we’re titling the window “Geekedu Song Lyric Extractor”. You’re free to name the project window whatever you’d like, but make sure the name is clear and easy to understand! Once this step is complete, the code should look like this:
Next, it’s time to create labels for the GUI, as well as create the text input box. We’ll start by creating a label to let users know what the program does. For this example, we will create a label that says “Enter Song Name:” so that users know where to enter the title of the song they want lyrics for. Next, we’ll add another label below that says “Result:” to let users know what the song’s lyrics are. The code for this step of the project will be:
There is a set format that is used for creating labels with the Tkinter GUI. The format is as follows:
Label(root, text = 'Add Label Text Here', bg= 'background colour').grid(row= row-number, column = column-number, sticky = compass-direction). Note that any section in bold will be replaced with the desired text, format, or coordinates for the label.
Hint: The “sticky” format option defines what happens if the button, object, or label is too big for the window. In this scenario, the label will be aligned to the West (or left). However, other options are available such as N, S, E, W, NE, NW, SE, and SW. Now that the labels for the GUI are complete, it’s time to add the text input box. To start, we’ll create a variable called “result”, and set it to a blank string variable.
Hint: This will let the program know that the text entered into the text box will be a string, and the computer can take this information and store it as a variable.
Next, we’ll create a text box where users can input a song title. This element will follow a very similar format to the labels we created earlier, and in the end, the code will look like this:
Once this step is complete, the main GUI is finished and it’s time to move on to code the main download function of the program.

Step 3: Define the Get Lyrics Function
Now we’ll move on to create the get lyrics function. This function will take the user’s input and use the lyrics_extractor API to display the lyrics. First, we’ll start by defining the function, then we’ll create a variable called “extractLyrics”. Then, we will set it equal to the songLyrics module, along with our API key and GCS engine ID.
Hint: API stands for Application Programming Interface, and is a way to allow two programs to “talk” to one another. GCS Engines are Google Custom Search engines and will allow you to search for information on the web within your program. You can find an API key by going to this website, and get a GCS engine by going to this website and inserting https://genius.com/ as a website to get search results from. Follow the instructions on the website, or look at the images below to see how to get your key:
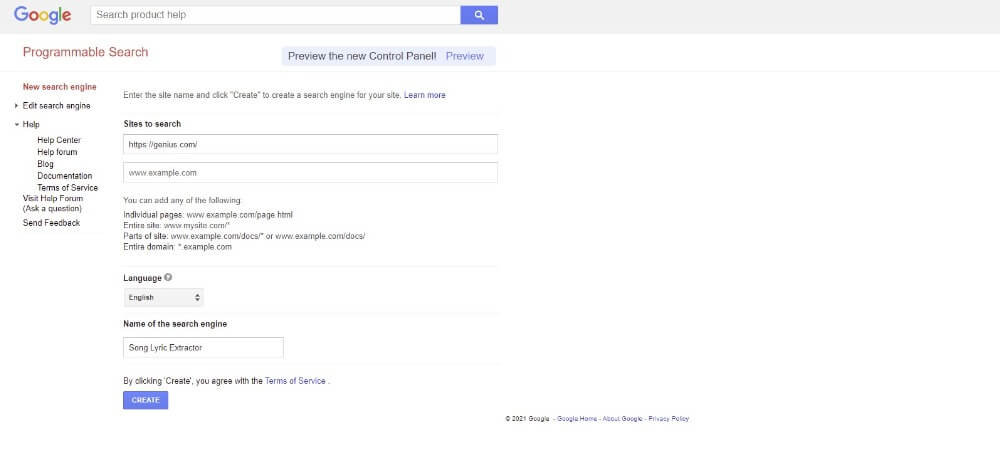

Once this is done, insert them into the next line of code like this:
Once this is complete, the first section of code for this step of the program will look like this:
Next, we’ll create a temporary variable called “temp”, and use it to store the resulting lyrics that the program gets from the inputted title. Then, all we need to do is create a variable called lyricsResult to hold this value. Finally, we’ll create a result variable and set it equal to lyricsResult so that the correct lyrics can be displayed for the user. The code for the rest of this section will be:
Step 4: Create the GUI’s ‘Download’ Button
Finally, we just need to add the ‘Show Lyrics’ button to the GUI, as well as a line of code to run the whole project. We will use the same basic formatting as was previously used for the labels and input box to create a button. Finally, we will use the root.mainloop() command to set up the program and allow it to be run. This command will essentially run the Python file and loop it forever, which means the program will always run. Once this final step is complete the code will look like this:
Note that in the line of code where we set up the Generate Answer button, the format is slightly different from that of the labels. This is because we added a command that will be run when the button is pressed. We have also used the .grid method of formatting the button. What this does is it aligns the button in row 0, column 5, then pads it with 5 pixels on all sides. Once this is finished, the code is complete and the project is finished!
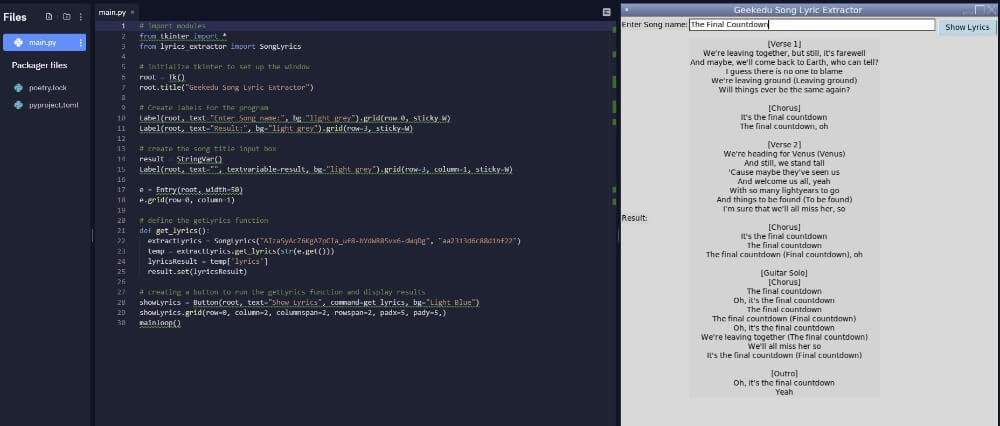
Project Complete!
And now the project is complete! At this point, you will be able to experiment with the song lyric extractor program and can learn all the lyrics to your favourite songs! Hopefully, you’ve enjoyed this intermediate-level Python tutorial for kids, and if you’re stuck or have any issues with your code, try reviewing it again either in your text editor or by looking at the code mentioned above as a reference.